Observer pattern
Today, I will try to present a observer pattern.It is part of behavioral patterns and is relatively simple so I think it’s worth it to present.
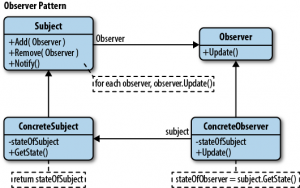
Subject – the object of which we want to obtain information
Observer – objects pending notification of the change in the observed object
When we use it?
When we have object(subject) that performs an operation and we want to inform other objects about the result of this operation. Then we can implement observer pattern.
I will show you how it works on the example.
Example
In our example will use price list as Subject. It will have users as observers and will inform them(users) about changing prices.
The first thing we need to do is to implement two interfaces Observer and Subject
public interface ISubject { int currentPrice { get; set; } void addObserver(IObserver o); void deleteObserver(IObserver o); void tellObserver(); }
Our interface ISubject be able to add, delete, notify observers and has a property with the current price.
public interface IObserver { void updateData(); }
Observer(listener) will update the data in our case the current price from price list.
Class PriceList looks like this:
public class PriceList : ISubject { //list of objects that will observe the objects of this class private List<IObserver> _listOfObservers = new List<IObserver>(); public int currentPrice { get; set; } public void addObserver(IObserver o) { _listOfObservers.Add(o); } public void deleteObserver(IObserver o) { _listOfObservers.Remove(o); } public void tellObserver() { foreach (var item in _listOfObservers) { item.updateData(); } } public int changePrice(int newPrice) { return currentPrice = newPrice; } }
Our class price list has list of object that will observe the object of this class.
Class User:
class User : IObserver { //private field for storing the current price private int _currentPrice; //reference to observed object private ISubject priceList; //user name private string _name; public User(string name, ISubject subject) { priceList = subject; _name = name; } public void updateData() { _currentPrice = priceList.currentPrice; Console.WriteLine("\nHey {0}, price has just changed to {1} PLN", _name, _currentPrice); } }
Class user receives two parameters User Name and references to the observed object. The method updateData displays the user’s name and the current price .
Therefore, let us see how our pattern works.
static void Main(string[] args) { PriceList pl = new PriceList(); User u1 = new User("Pawel",pl); User u2 = new User("Sabina",pl); // adding observers pl.addObserver(u1); pl.addObserver(u2); // change/set current price pl.changePrice(20); pl.tellObserver(); // remove one of observers and change price pl.deleteObserver(u2); pl.changePrice(15); pl.tellObserver(); Console.ReadKey(); }
Result:
Summary
As we have seen implementation observer patter is simple. If we think about it, this pattern may have a lot of applications.
Link to the project : HERE